In this article, we discuss about how to write factorial programs in java and javascript,using for loops, while loops & arrays.
Introduction
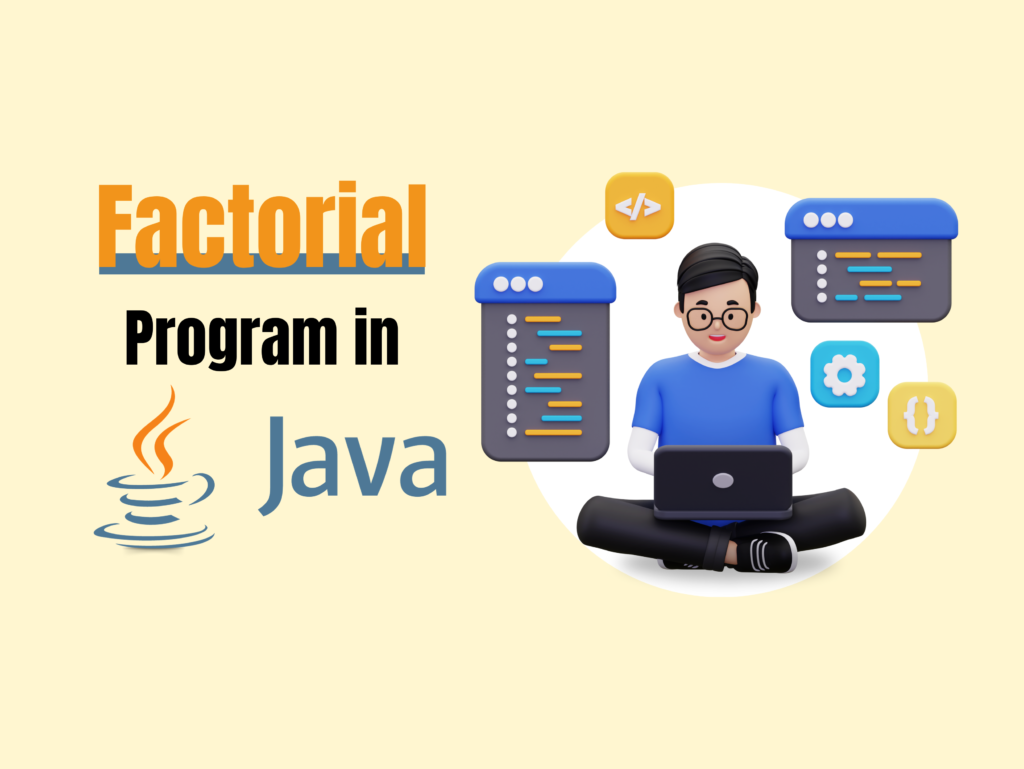
Factorial calculations are a fundamental concept in mathematics and programming. Whether you are working with Java or JavaScript, understanding how to write factorial programs is essential. We will examine several approaches to building factorial programs in Java and JavaScript in this article. Basic factorial programs, recursive techniques, while and for loops, and even an array-based strategy will all be covered. You will have a firm understanding of factorial computations in these two flexible languages by the end of this article.
A like blog – Pictory AI Complete Guide
Factorial Program in Java
Let’s start with a simple factorial program in Java. Here’s the code snippet:
public class FactorialJava {
public static int factorial(int n) {
if (n == 0) {
return 1;
}
return n * factorial(n – 1);
}
public static void main(String[] args) {
int number = 5; // Change this to calculate the factorial for a different number
int result = factorial(number);
System.out.println(“Factorial of ” + number + ” is ” + result);
}
}
This Java program calculates the factorial of a number using recursion.
Factorial Program Using For Loop in Java
For those who prefer an iterative approach, here’s a Java program using a for loop:
public class FactorialJavaForLoop {
public static int factorial(int n) {
int result = 1;
for (int i = 1; i <= n; i++) {
result *= i;
}
return result;
}
public static void main(String[] args) {
int number = 5; // Change this to calculate the factorial for a different number
int result = factorial(number);
System.out.println(“Factorial of ” + number + ” is ” + result);
}
}
Factorial Program in Java Using a While Loop
If while loops are more to your liking, you can implement the factorial program like this:
public class FactorialJavaWhileLoop {
public static int factorial(int n) {
int result = 1;
while (n > 0) {
result *= n;
n–;
}
return result;
}
public static void main(String[] args) {
int number = 5; // Change this to calculate the factorial for a different number
int result = factorial(number);
System.out.println(“Factorial of ” + number + ” is ” + result);
}
}
Factorial Program in JavaScript
Moving on to JavaScript, here’s a factorial program using recursion:
function factorial(n) {
if (n === 0) {
return 1;
}
return n * factorial(n – 1);
}
const number = 5; // Change this to calculate the factorial for a different number
const result = factorial(number);
console.log(`Factorial of ${number} is ${result}`);
Factorial Program Using a While Loop in JavaScript
For those who prefer while loops in JavaScript, here’s an implementation:
function factorial(n) {
let result = 1;
while (n > 0) {
result *= n;
n–;
}
return result;
}
const number = 5; // Change this to calculate the factorial for a different number
const result = factorial(number);
console.log(`Factorial of ${number} is ${result}`);
Factorial Program in JavaScript Using an Array
To showcase the versatility of JavaScript, here’s a factorial program using an array:
function factorial(n) {
let result = 1;
const numbers = Array.from({ length: n }, (_, i) => i + 1);
for (const number of numbers) {
result *= number;
}
return result;
}
const number = 5; // Change this to calculate the factorial for a different number
const result = factorial(number);
console.log(`Factorial of ${number} is ${result}`);
Conclusion
In this article, we looked at a number of methods—such as recursion, for loops, while loops, and even an array-based approach—for creating factorial programs in Java and JavaScript. Knowing how to compute factorials in various languages is a useful ability, regardless of your level of programming experience. Work on these programs, try out various numbers, and improve your programming skills.
A like blog – Kaiber AI Video Editing Tool